Theme switching with SSR and SSG
By leveraging Static Site Generation (SSG) in our documentation site, we optimize performance by serving pre-rendered pages. However, this approach introduces challenges with theme switching, such as flickering due to mismatches between the pre-rendered HTML and client-side theme preferences.
To resolve this, we implemented a data-theme
attribute combined with CSS variables, ensuring that the correct theme is applied before React hydrates the page. This method prevents the flash effect and provides a seamless user experience. Additionally, our implementation in _document.tsx
and _app.tsx
, along with the ThemeProvider
configuration, ensures that theme switching is handled efficiently without unnecessary re-renders.
By following this approach, we maintain both the performance benefits of SSG and a smooth, flicker-free theme transition for users.
Server-Side Rendering (SSR) and Static Site Generation (SSG) are two different rendering strategies used in frameworks like Next.js, each with its own advantages and use cases.
SSR generates pages on the server for every request, ensuring that users always receive the latest data. In Next.js, this is achieved using getServerSideProps
. This approach is useful for dynamic content that changes frequently, such as personalized dashboards or real-time data feeds. However, because the server must generate the page before sending it to the client, it can be slower than other rendering methods.
SSG, on the other hand, generates pages at build time, meaning the content is pre-rendered and stored as static files. In Next.js, this is done using getStaticProps
. This method is ideal for pages where content doesn’t change often, such as blogs, marketing pages, or documentation. Since the pages are served as static files, they load much faster than SSR-generated pages.
As our docs site data doesn't change between builds we use SSG to generate our pages to utilize the speed benefits of static site generation.
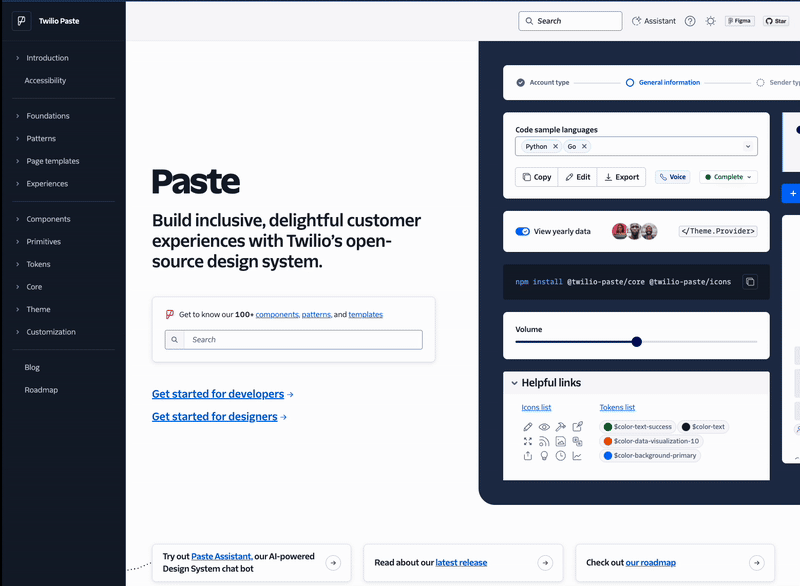
When loading our site previously with a dark mode preference you would see the above flicker. This is due to the style being applied at the JavaScript level on the client and the server rendering the default light theme. This means there is a brief moment where the light theme is applied before the dark theme is applied by JavaScript.
When using SSG (Static Site Generation) and SSR (Server-Side Rendering) in Next.js, you may sometimes experience a flashbang effect or theme flickering when loading a page. This happens due to differences in how the initial HTML is generated versus how the React client-side hydration process takes over, recognizing the client preferences. Here’s why:
With SSG, the page is pre-built at build time and served as static HTML. If the theme (e.g., dark mode or light mode) is determined dynamically on the client side—perhaps by checking localStorage or user preferences—there can be a mismatch between the initial HTML (which doesn't know the user's preference) and the hydrated React state. This causes a flicker when React updates the page with the correct theme after loading.
SSR generates the page on each request, which can help deliver the correct theme initially, but if the theme is still set on the client side, the same flickering issue can occur.
Next.js sends pre-rendered HTML first, then React hydrates it by attaching event listeners and making it interactive. If the theme is set dynamically via JavaScript on the client, React may initially render the wrong theme (based on the server-provided HTML) before correcting it after hydration. This is particularly noticeable when using a system preference-based theme (like dark mode) or when checking user preferences stored in localStorage.
If you don’t handle the theme properly, Next.js may serve a default theme (e.g., light mode) before applying the correct one. This results in a brief flash where the wrong theme appears before React updates it.
Using CSS variables with data-theme
set on the html
or body
is the most effective solution as the styles are applied before React hydrates the page. This ensures that the correct theme is applied from the start, preventing any flickering or flash.
This works because the browser applies the correct theme instantly, even before JavaScript runs, ensuring there is no mismatch between the server-rendered page and the client-rendered one. The original behavior picked up stlying changes after JavaScript executes, causing a visible flicker when the correct theme is applied later.
To support this approach, as of @twilio-paste/design-tokens v10.14.0 and @twilio-paste/core v20.23.0, we have added a new file to each of our themes called tokens.data-theme.css
which contains the CSS variables for each theme when the data-theme
is set.
An example of how this CSS file is structured:
@twilio-paste/design-tokens/dist/themes/twilio-dark/tokens.data-theme.css
body[data-theme="twilio-dark"] {
--color-background-user: rgb(34, 9, 74);
--color-background-notification: rgb(214, 31, 31);
--color-background-trial: rgb(5, 41, 18);
--color-background-subaccount: rgb(18, 28, 45);
--color-background-primary-stronger: rgb(204, 228, 255);
...
We have also added a new prop to the ThemeProvider
called useCSSVariables
which will allow you to use CSS variables instead of the theme files we previously configured. Note that we only want to use CSS variables when encoutnering this issue and not as a standard. For all other use cases we recommend using the theme
prop.
This section will cover how we implemented this solution on the Paste documentation site using Next.js.
We added a script to the head
of our _documents.tsx
file to set the data-theme
attribute on the body
element using a script. This script will check to see if users have a cookie set and if not check the system preferences to determine whether users prefer dark or light themes, and apply the dark theme if they do. Otherwise we do not set a value. You will see in _app.tsx
how we handle the default value.
Here is the script that is found in _documents.tsx
:
Setting data-theme using cookie before render
<script
type="text/javascript"
dangerouslySetInnerHTML={{
__html: `
let parts = typeof document !== "undefined" && document?.cookie.split("paste-docs-theme=");
let cookie = parts.length == 2 ?parts?.pop().split(";").shift() : null;
if(cookie){
document.body.dataset.theme = cookie;
}
else if(window !== "undefined" && window.matchMedia && window.matchMedia("(prefers-color-scheme: dark)").matches){
document.body.dataset.theme = "twilio-dark";
}
`,
}}
/>
In our _app.tsx
file we import the CSS files that should be applied. We have 2: One that applies the values to root and is considered the default theme, and the other that uses the new tokens.data-theme.css
attribute to apply the theme based on the value set in the data-theme
attribute.
You can find the source code for _app.tsx here.
Importing CSS files
import "@twilio-paste/design-tokens/dist/themes/twilio-dark/tokens.data-theme.css";
import "@twilio-paste/design-tokens/dist/themes/twilio/tokens.custom-properties.css";
Note here that the import "@twilio-paste/design-tokens/dist/themes/twilio/tokens.custom-properties.css"
is the default theme and the "@twilio-paste/design-tokens/dist/themes/twilio-dark/tokens.data-theme.css"
is the theme that is applied when the data-theme
attribute is set to twilio-dark
.
In this same file we set the ThemeProvider to use CSS variables instead of the theme files we previously configured. Note that we only want to use CSS variables when encountering this issue and not as a standard. For all other use cases we recommend using the theme
prop.
ThemeProvider CSS implementation
<Theme.Provider
useCSSVariables={true}
cacheProviderProps={{ key: "next" }}
>
ThemeProvider theme implementation
<Theme.Provider
theme={theme || 'twilio'}
cacheProviderProps={{ key: "next" }}
>
We have a hook that is used to switch the themes in useDarkMode.tsx
. In the setMode
function, we handle setting the data attribute on the body element. This occurs when the user intentionally changes the theme using the theme switcher in the header. As this will run client side, it cannot be used to set the default value. That comes from the script in the _document.tsx
.
setMode
const setMode = (mode: ValidThemeName): void => {
setCookie(null, "paste-docs-theme", mode, { path: "/" });
document.body.dataset.theme = mode;
setTheme(mode);
};
As this update the body attribute theme
and we are importing a CSS stylesheet that switches the variable values based on that value, the new theme will be applied. This works because the ThemeProvider
is listening for the variable values and not pulling them from a static theme via JavaScript. It also stores the preference in a cookie ready to be picked up and applied to the body before React hydrates the page resulting in no flicker.